Build
2 min read
- Authors
- Name
- Karan Pratap Singh
- @karan_6864
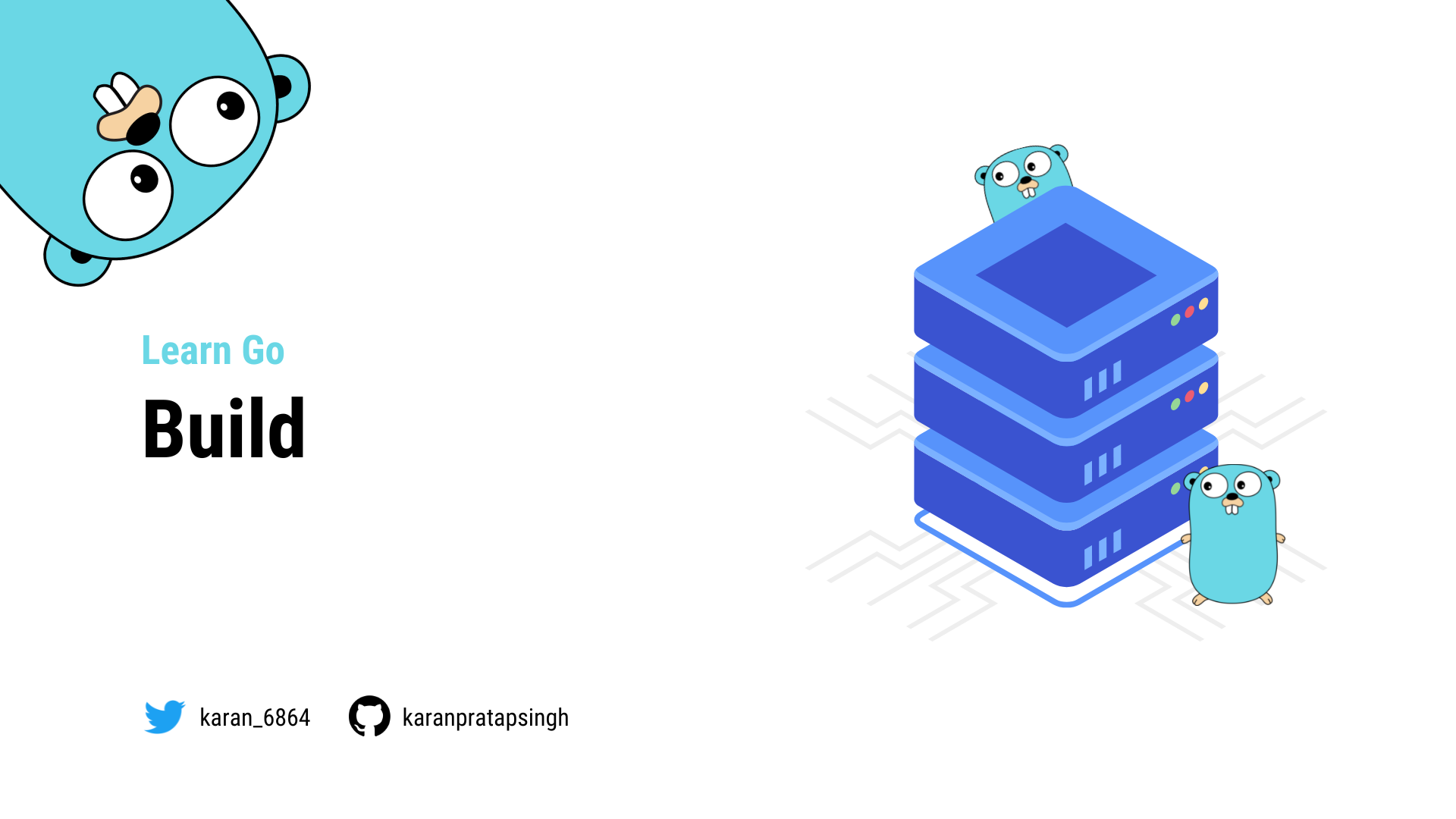
Building static binaries is one of the best features of Go which enables us to ship our code efficiently.
We can do this very easily using the go build
command.
package main
import "fmt"
func main() {
fmt.Println("I am a binary!")
}
$ go build
This should produce a binary with the name of our module. For example, here we have example
.
We can also specify the output.
$ go build -o app
Now to run this, we simply need to execute it.
$ ./app
I am a binary!
Yes, it's as simple as that!
Now, let's talk about some important build time variables, starting with:
GOOS
andGOARCH
These environment variables help us build go programs for different operating systems and underlying processor architectures.
We can list all the supported architecture using go tool
command.
$ go tool dist list
android/amd64
ios/amd64
js/wasm
linux/amd64
windows/arm64
.
.
.
Here's an example for building a windows executable from macOS!
$ GOOS=windows GOARCH=amd64 go build -o app.exe
CGO_ENABLED
This variable allows us to configure CGO, which is a way in Go to call C code.
This helps us to produce a statically linked binary that works without any external dependencies.
This is quite helpful for, let's say when we want to run our go binaries in a docker container with minimum external dependencies.
Here's an example of how to use it:
$ CGO_ENABLED=0 go build -o app